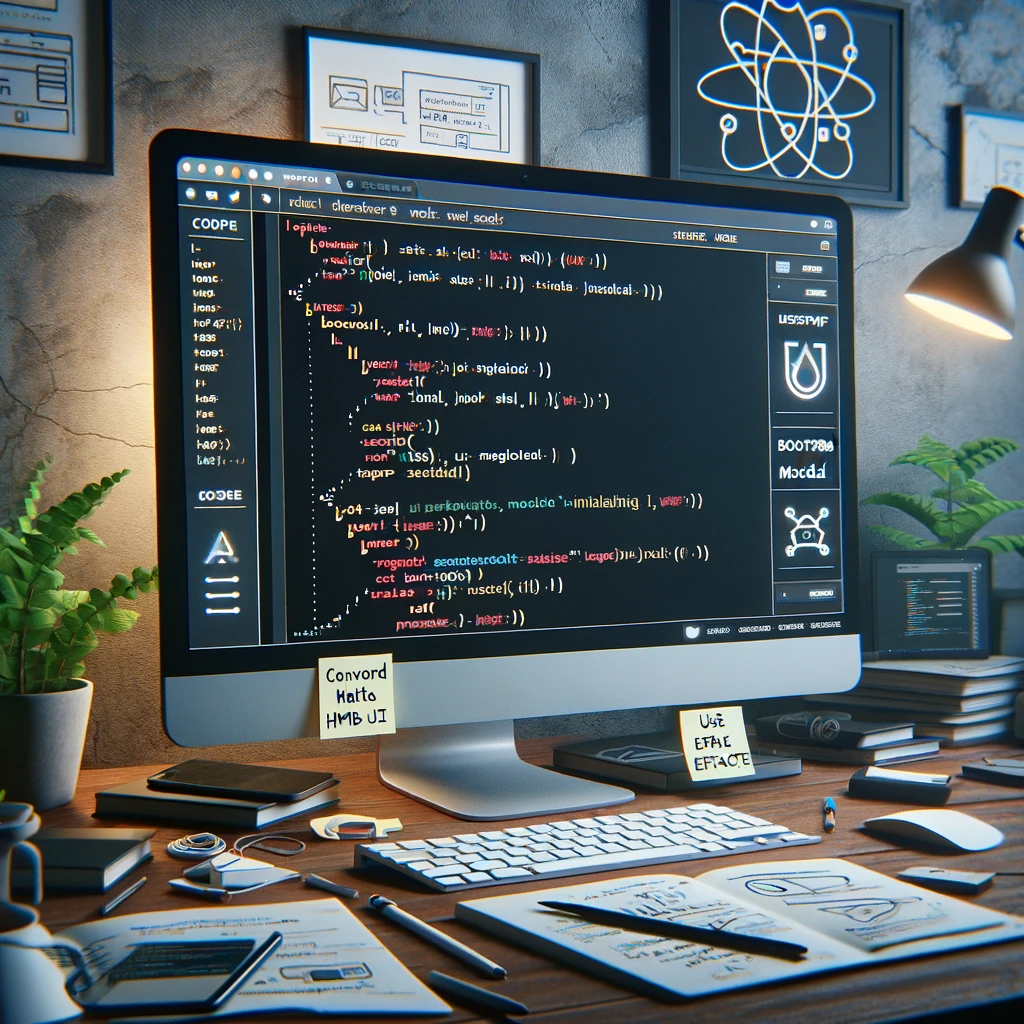
When integrating Bootstrap modals into a React application, there are several potential issues to consider, especially if you’re transitioning from a static HTML page to a React component. Here’s a step-by-step guide to help you troubleshoot and understand why your Bootstrap modal might not be working in your React application:
Step 1: Check Bootstrap and jQuery Integration
- Bootstrap Dependency: Ensure that Bootstrap and its dependencies (like jQuery, if you’re using Bootstrap versions prior to 5) are correctly integrated into your React project. For Bootstrap 5 and later, jQuery is not needed.
- React Bootstrap: Consider using React-Bootstrap, which re-implements Bootstrap components as React components, removing the need for jQuery.
Step 2: Import Bootstrap CSS
- Make sure that the Bootstrap CSS is correctly imported in your React component or in your main app file. You can import it directly from
node_modules
if you’ve installed Bootstrap via npm/yarn, or use a CDN link.
import 'bootstrap/dist/css/bootstrap.min.css';
Step 3: Initialize Bootstrap JavaScript (If Needed)
- If your modals rely on Bootstrap’s JavaScript (Bootstrap versions prior to 5), ensure it’s correctly initialized. In a React context, you might need to initialize Bootstrap components manually in a
useEffect
hook when the component mounts.
Step 4: Convert Static HTML to React Components
- JSX Syntax: Convert your HTML to JSX syntax. This includes changing
class
toclassName
,for
tohtmlFor
, and ensuring all tags are properly closed. - Event Handling: Ensure that any event handlers (like onClick for modal triggers) are correctly implemented in React. Use React’s event handling methods instead of inline JavaScript or jQuery.
Step 5: Manage Modal State in React
- State Management: Use React’s state management to control the opening and closing of the modal. You can use
useState
to hold the modal’s open/close state. - Controlled Component: Turn your modal into a controlled component, where its visibility is controlled by React state.
const [showModal, setShowModal] = useState(false);
// Open modal
const handleOpen = () => setShowModal(true);
// Close modal
const handleClose = () => setShowModal(false);
Step 6: Implement React Component for Modal
- Create a React component for the modal and control its visibility using the state from the parent component.
- Pass
showModal
andhandleClose
as props to this component.
Step 7: Debugging
- Console Logs: Use console logs to ensure that click events are firing and state is changing as expected.
- React Developer Tools: Use React Developer Tools to inspect the component tree and state.
Step 8: Check for Conflicts
- CSS/JS Conflicts: Ensure there are no conflicts between Bootstrap’s JavaScript/CSS and your React application.
Conclusion
Integrating Bootstrap modals into a React application often requires some adjustments to account for React’s component-based architecture and state management. By following these steps, you should be able to identify and resolve the issue with your Bootstrap modals in React. Remember, React’s way of handling DOM and events is different from how it’s done in plain HTML and JavaScript, and adapting to this difference is key in solving such integration issues.
–
–
Tell me more step by step about Bootstrap modal works in static webpage, but not in React.js equivalent
I’m building a site from a Bootstrap template that comes with some modal components, specifically in the ‘portfolio’ section of this template. The modals work fine when my page is entirely front end. But when I copy and paste the page out of index.html and into a React component to mount, nothing happens upon clicking upon any modal trigger.
There are no errors or warnings. Is there a simple explanation for what I’m missing for why the page isn’t behaving the same? Thanks in advance.</p
Troubleshooting Bootstrap Modals in a React.js Environment
When using Bootstrap modals in a static webpage, they function as expected and present no issues. However, when the same webpage is incorporated into a React.js component and mounted, the modals fail to work upon clicking the trigger. This issue occurs despite the absence of any error messages or warnings. The reason for this discrepancy lies in the difference in how static webpages and React.js handle DOM manipulation and event binding. In a static webpage, the modal functionality is immediately available due to the direct inclusion of the Bootstrap JavaScript and CSS. However, in a React environment, additional steps are required to ensure that the modal triggers and functionality are properly integrated with React’s virtual DOM. This can involve using React’s ref system to directly interact with the modal elements or utilizing third-party libraries such as react-bootstrap. By addressing these differences and integrating the modal components properly within the React structure, the issue with the modals not working should be resolved.
Keyword 1: Bootstrap Modals, Keyword 2: React.js Environment
Keyword 1 Explained: Bootstrap modals refer to the interactive pop-up dialog boxes provided by the Bootstrap framework, which are used to display content, forms, or other UI elements on a web page. They are triggered by user actions such as button clicks and are commonly used for displaying additional information or interactive components.
Keyword 2 Explained: A React.js environment pertains to the ecosystem and structure in which React, a JavaScript library for building user interfaces, operates. It involves the use of components, virtual DOM, and a unidirectional data flow to create dynamic web applications. React.js introduces a different approach to handling DOM manipulation and event binding compared to traditional static webpages.
Enhance Your Bootstrap Modals in React.js
Understanding the nuances of integrating Bootstrap modals in a React.js environment can significantly enhance your web development experience. By overcoming the differences in DOM manipulation and event handling, you can seamlessly leverage the power of Bootstrap modals within your React applications, adding a layer of interactivity and functionality to your web projects. Explore how to integrate these modal components effectively and take your React.js development to the next level.
답글 남기기 응답 취소